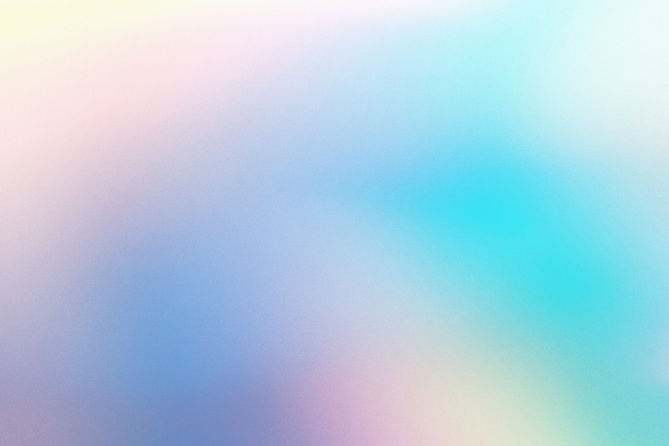
Room Temp Controller
Arduino input
01
DESCRIPTION & MATERIALS
This is an automated room temperature controller to adjust the temperature of the room. Uses input from the temperature sensor.
If temperature reading is above desired range, LCD displays "temp is HIGHER" and the FAN (dc motor) is turned on. If the temperature is below the desired range LCD display "temp is LOWER" and the HEATER (LED)
is turned on. Once the temperature is in the desired range the LCD displays "Temp is Normal", "Turn off all" and both the HEATER and FAN
are turned off.
Otherwise, if an error has occurred the LCD will display
"Something went wrong in ckt". we will need:
1 arduino Uno R3
1 LCD 16*2 screen
1 250kiloOhms Potientiometer
3 220Ohms Resistor
1 Diode
1 green LED
1 temperature sensor
1 DC motor
1 NPN Transistor
02
CODES
#include <LiquidCrystal.h>
const int temp_trans_pin = A0, Heater_pin = 13, FAN_pin = 6;
//Sets the desired Temp range between 15 and 20
float MinTemp =15, MaxTemp = 25;
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() {
lcd.begin(16, 2);
pinMode(Heater_pin, OUTPUT);
pinMode(FAN_pin, OUTPUT);
//Displays the desired Temp range
lcd.print("Room temp(C):");
lcd.setCursor(2,1);
lcd.print(MinTemp);
lcd.print("-");
lcd.print(MaxTemp);
delay(2000);
}
void loop() {
float Eqv_volt, SensorTemp;
//Reads the volatge and converts it to Temp
Eqv_volt = analogRead(temp_trans_pin) * 5.0 / 1023;
SensorTemp = 100.0 * Eqv_volt-50.0;
//Displays the Sensor Reading
lcd.clear();
lcd.print("Sensor Reading:");
lcd.setCursor(2, 1);
lcd.print(SensorTemp);
lcd.print("C");
delay(2000);
//Compares the reading to the desired temp
if(SensorTemp > MaxTemp){
lcd.clear();
lcd.print("temp is HIGHER!");
//Turns on the FAN(dc motor)
lcd.setCursor(0, 1);
lcd.print("Turn on FAN!");
for( int i = 0; i <= 255; i++){
analogWrite(FAN_pin, i);
}
delay(3000);
lcd.clear();
lcd.print("Now temp is OK!");
lcd.setCursor(0, 1);
lcd.print("Turn off FAN");
//Turns off FAN slowly
for( int i = 255; i >= 0; i--){
analogWrite(FAN_pin, i);
}
delay(2000);
}
else if(SensorTemp < MinTemp){
lcd.clear();
lcd.print("temp is LOWER!");
lcd.setCursor(0, 1);
lcd.print("Turn on HEATER!");
//Turn on Heater(LED)
digitalWrite(Heater_pin, HIGH);
delay(3000);
lcd.clear();
lcd.print("Now temmp is OK!");
lcd.setCursor(0, 1);
lcd.print("Turn off HEATER!");
delay(1000);
digitalWrite(Heater_pin, LOW);
lcd.clear();
}
//Temp is in perfect range so devices turned off
else if(SensorTemp > MinTemp && SensorTemp < MaxTemp){
lcd.clear();
lcd.print("Temp is NORMAL");
lcd.setCursor(2, 1);
lcd.print("Turn off all!");
delay(1000);
lcd.clear();
}
//Something went wrong in the circuit
else {
lcd.clear();
lcd.print("Something went");
lcd.setCursor(2, 1);
lcd.print("WRONG in the ckt");
delay(1000);
lcd.clear();
}
delay(1000);
}
03
OUTPUT
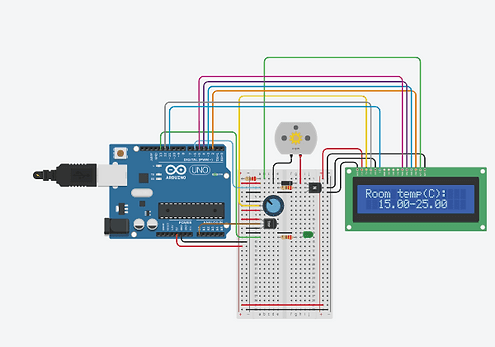
04
ANALYSIS
The system run smoothly and without fault.